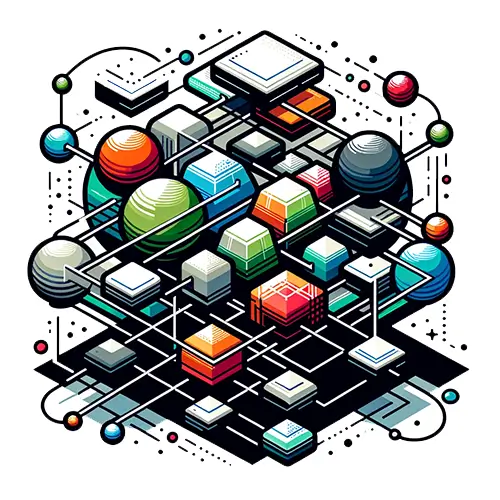
Object-Oriented Programming (OOP) is a paradigm in software engineering that models complex software systems as interactive objects. This approach, deeply rooted in the real-world metaphor, emphasizes software organized into discrete units (classes and objects) that encapsulate state and behavior.
Fundamental Concepts
Fundamental concepts include encapsulation, polymorphism, and inheritance, collectively contributing to a structured and intuitive approach.
Objects & Classes
Objects: Instances of classes representing specific entities with attributes, behavior, and data.
Classes: Blueprints for objects, defining their structure and behavior.
class Car {
String model;
int year;
void displayInfo() {
System.out.println(model + " " + year);
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.model = "Toyota";
myCar.year = 2021;
myCar.displayInfo();
}
}
Encapsulation
Bundling data and methods into classes and restricting access to an object’s components.
class Person {
private String name;
public void setName(String newName) {
name = newName;
}
public String getName() {
return name;
}
}
Inheritance
Allowing new classes to inherit properties and methods from existing classes.
class Vehicle {
void run() {
System.out.println("Vehicle is running");
}
}
class Car extends Vehicle {
void run() {
System.out.println("Car is running safely");
}
}
Polymorphism
Enabling objects of different types to respond to the same method calls in their own way.
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
Abstraction
Focusing on essential qualities of an object and hiding unnecessary details.
abstract class Animal {
abstract void makeSound();
}
class Cat extends Animal {
void makeSound() {
System.out.println("Meow");
}
}
Advanced Concepts
Beyond the basics, OOP encompasses advanced techniques like abstraction, design patterns, and SOLID principles. These concepts enhance code’s scalability, maintainability, and efficiency, addressing complex software development challenges.
Association, Aggregation, and Composition:
Association is the most general form, representing a link between objects where they interact but do not have ownership over each other, like the relationship between a teacher and a student. Aggregation is a more specific form of association, signifying a “has-a” relationship with a whole/part dynamic, such as a car having an engine, where the engine can exist independently of the car. Composition is the strictest form, also a “has-a” relationship but with a dependent nature, where the life of the ‘part’ is controlled by the ‘whole’, exemplified by the relationship between a house and its rooms. These concepts are essential in modeling complex systems in OOP, reflecting the varying degrees of dependency and association between different objects.
class Engine {
// Engine details
}
class Car {
private Engine engine;
Car() {
engine = new Engine();
}
}
Interfaces & Abstract Classes
Interfaces and abstract classes are used to define contracts for implementation, but in different ways. Interfaces are akin to a template, consisting solely of abstract methods that must be implemented by any class that ‘implements’ the interface, allowing for multiple inheritance as classes can implement multiple interfaces. This is ideal for defining a standard set of behaviors across different classes with diverse implementations. Abstract classes, in contrast, are base classes that cannot be instantiated directly and can contain a mix of implemented and abstract methods, along with member variables and constructors. They are used when various classes share common functionalities and states, allowing subclasses to inherit a default implementation and reduce code redundancy. Thus, while interfaces are used for establishing a common contract without implementation, abstract classes provide a partial blueprint with some shared functionality for subclasses.
interface Vehicle {
void run();
}
class Car implements Vehicle {
public void run() {
System.out.println("Car is running");
}
}
Design Patterns & SOLID Principles
Design Patterns and SOLID Principles are key concepts that guide the development of robust and maintainable software. Design Patterns are standardized solutions to common problems in software design, offering templates like Singleton, Observer, and Factory for code flexibility and reusability. SOLID Principles, comprising Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion, are guidelines that promote clean code through principles like modularity, abstraction, and decoupling. Together, Design Patterns provide practical methods to tackle frequent design challenges, while SOLID Principles establish foundational rules for writing adaptable and efficient code, ensuring that OOP systems are well-structured and future-proof.
class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Technical Proficiency & Best Practices
Effective Object-Oriented Programming (OOP) demands a firm grasp of its fundamental principles—encapsulation, inheritance, abstraction, and polymorphism. This proficiency ensures the creation of systems with well-defined, modular classes and objects that encapsulate specific functionalities and data. Continue your focus by learning:
- Implementing OOP Principles
- Applying Design Patterns and SOLID Principles
- Refactoring and Optimization
Historical Context & Evolution
Over the years, these languages and others have evolved, incorporating functional programming elements and enhancing capabilities for complex systems, reflecting OOP’s adaptability and enduring relevance in software development.
1960’s
1970’s
1980’s
1990’s
Interconnections & Influences
OOP’s influence extends to other paradigms, such as functional programming, where concepts like immutability are blended with object-oriented approaches. Similarly, OOP has been adapted in response to trends in software development, leading to hybrid models.
- OOP contrasts with Procedural Programming by emphasizing objects rather than procedures or functions.
- Concepts from OOP, like encapsulation and polymorphism, have influenced Multi-Paradigm and Aspect-Oriented Programming.
- Often used alongside Functional Programming in languages like Scala, it blends object-oriented concepts with functional paradigms for more robust solutions.
Case Studies & Real-World Examples
In real-world scenarios, OOP demonstrates its versatility. Java-based Android app development and Unity game development are prime examples of OOP’s principles facilitating complex software solutions.
- Java-based Android app development utilizes OOP for creating apps with classes representing UI elements and backend functionalities.
- Game Development in Unity leverages C for scripting game behavior and environment using OOP principles.
Common Misconceptions & Clarifications
Common misconceptions, such as OOP is inherently slower or less efficient, are often based on misunderstandings. When correctly implemented, OOP offers robust and efficient solutions suitable for many applications.
- OOP is not inherently inefficient and slow.
- Efficiency depends more on design and implementation, not the Paradigm itself.
Recommended Learning Resources
Books and online courses offer practical and theoretical insights into OOP.
- Amazon Book: Object Oriented Analysis & Design
Future Trends & Evolving Aspects
OOP continues to evolve, adapting to modern software needs. Trends like increased integration with functional programming and using OOP in emerging technologies signify its ongoing relevance.
- Combined Functional Programming for more robust and maintainable code.
- Increased Use in IoT Applications where objects represent physical devices and sensors.
Skills & Career Pathways
Proficiency in OOP opens numerous career pathways, including software development, system design, and game development. Skills like class design, understanding of polymorphism, and application of design patterns are highly valued in the tech industry.
- Strengthen your understanding of encapsulation, inheritance, and polymorphism.
- Software development, game development, and systems engineering are several career pathways.
Practical Applications & Industry Relevance
OOP finds extensive applications across various domains, from creating robust enterprise software to developing engaging video games. Its principles are integral in areas requiring modular and scalable software solutions.
Software Development Domains
- OOP is crucial in web and mobile app development, game development, and enterprise solutions.
Real-World Problem Solving
- OOP’s real-world metaphor is adept at modeling complex entities and solving intricate problems.
Take Away
Object-oriented programming is a cornerstone of modern software development, offering a structured approach to building complex systems. Understanding its principles, patterns, and practices is essential for crafting robust, maintainable, and efficient software solutions. This comprehensive understanding enables leveraging OOP’s potential in creating versatile, real-world applications.